Upsell - dialog
This use case shows how to add an upsell or optional article pop-up to your Ticketing widget.
Example case
The example case is a parking ticket. The vendor wants to upsell a parking ticket when a customer tries to checkout without a parking ticket.
Note
It is possible to add timeslot articles in the pop-up.
Demo
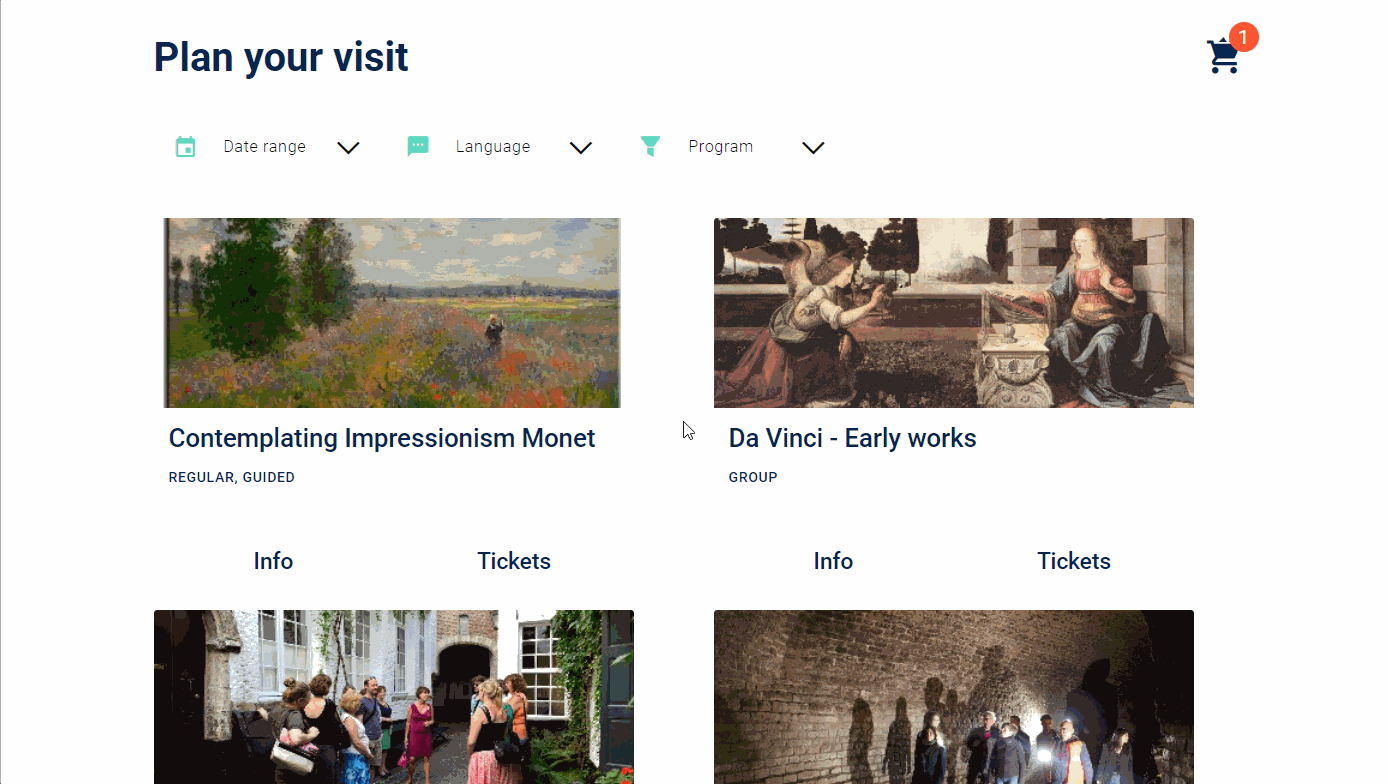
Pre-requisites
The parking ticket has been configured as an offer in Enviso Sales, and is fully set up with a ticket and unlimited capacity.
To find the ID of your offer, go to Sales > My offers. Select the offer. In the URL of the offer details page, the digits after detail/ is the offer ID.
simply go to the offer detail in the sales application and find it in the URL of the page. The ticket ID can be found in the response of the network request for that same page under products/id.
To find the ticket ID, go to Sales > Tickets.
Step-by-step guide
In order to implement the upsell popup, follow these steps:
Add the HTML to the body of your page. This uses the
enviso-dialog
component, to show a popup on the page.<enviso-dialog id="custom-parking-dialog"> <header class="enviso-dialog-header"> <div class="enviso-dialog-title">Don't you need a parking ticket?</div> <div class="enviso-dialog-description">Add it to your basket now!</div> </header> <enviso-button onClick="addParkingTicket()"></enviso-button> <enviso-button onClick="hideUpsellDialog()"></enviso-button> </enviso-dialog>
Define the
parkingOfferId
andparkingProductId
in the script.const parkingOfferId = *your offer ID*;const parkingProductId = *your ticket ID*;
Add an event-listener on step-changed to the script, so you know when you are navigating away from the basket. This is the time to shop the upsell popup. All helper functions used here can be found below in step 5.
document.addEventListener('step-changed', function (e) { // only show the dialog if the parking ticket is not yet bought if (isOfferInBasket(parkingOfferId)) return; // check the step in which the widget currently is; show the dialog only if the checkout has been clicked // and the enviso-basket-my-info is on the page let inCheckout = e.detail.activeStep.querySelector("enviso-basket-my-info"); if (inCheckout != null) { showUpsellDialog(); } }); //Add and event-listener on reservationcreated, so you can close the dialog if the items were added from the dialog. document.addEventListener('reservationscreated', function (e) { hideUpsellDialog(); });
Add the
addParkingTicket
function to the script.async function addParkingTicket() { await enviso.apis.directSelling.createReservations( { reservations: [ { productId: parkingProductId, quantity: 1 }]}); await enviso.basket.plugins.flatMap(plugin => plugin.refresh()); hideUpsellDialog(); }
Add the Helper functions to the script.
function isOfferInBasket(offerId) { if (!enviso.basket) return false; return enviso.basket.getBasketItemsArray().some(function(item) { return item.offerId === offerId }); } function showUpsellDialog() { document.querySelectorAll("#custom-parking-dialog")[0].show(); } function hideUpsellDialog() { document.querySelectorAll("#custom-parking-dialog")[0].hide(); }