Upsell - notice
This use case shows how to add a notice for upsell or optional article to your Ticketing widget.
Example case
The example case is a parking ticket. The vendor wants to upsell a parking ticket when a customer tries to checkout without a parking ticket.
Note
It is possible to add timeslot articles in the pop-up.
Demo
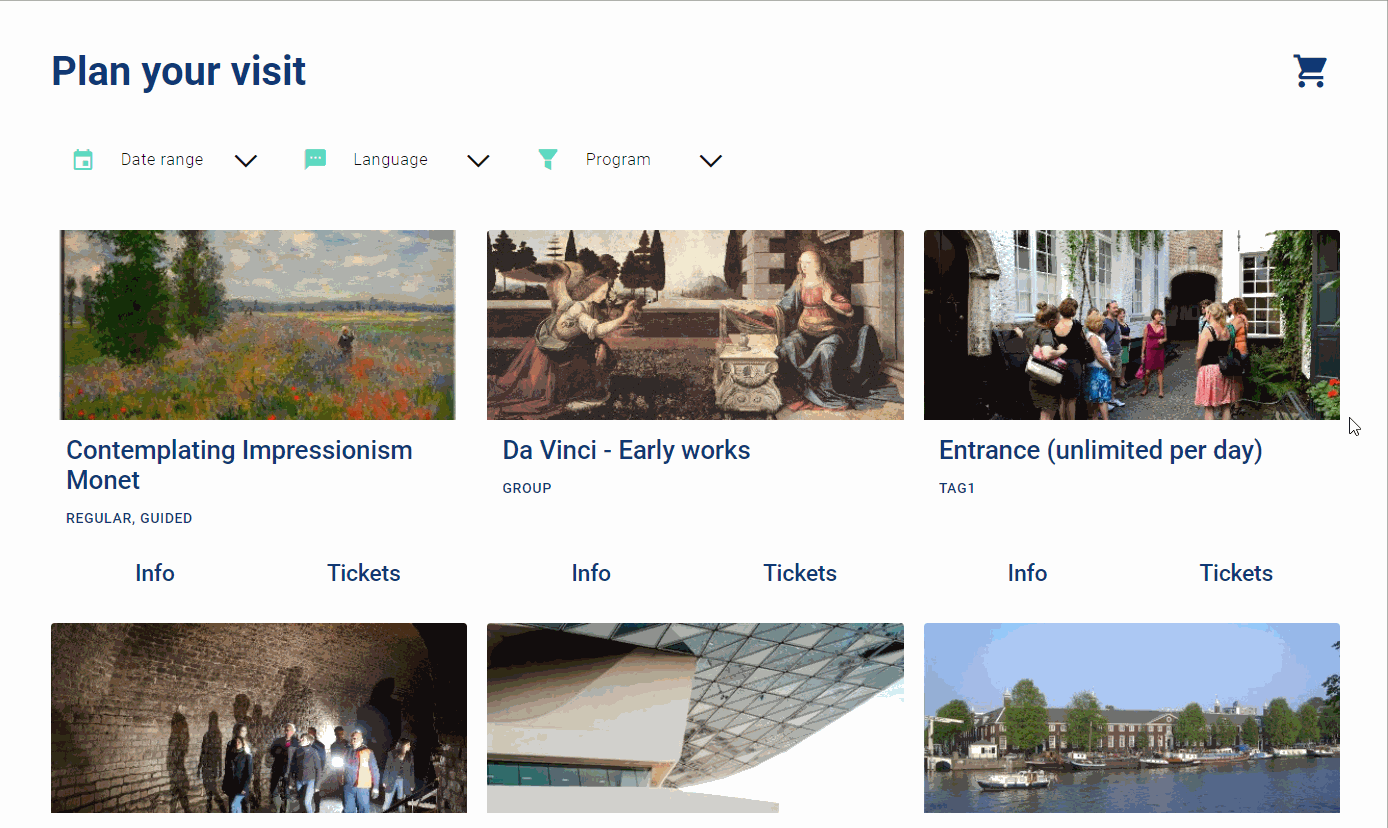
Pre-requisites
The parking ticket has been configured as an offer in Enviso Sales, and is fully set up with a ticket and unlimited capacity.
To find the ID of your offer, go to Sales > My offers. Select the offer. In the URL of the offer details page, the digits after detail/ is the offer ID.
simply go to the offer detail in the sales application and find it in the URL of the page. The ticket ID can be found in the response of the network request for that same page under products/id.
To find the ticket ID, go to Sales > Tickets.
Step-by-step guide
Add the HTML to the body of your page.
This example uses the
enviso-notice
to provide you with a fast and nice styling. Note theonclick
event on the button, to handle the behaviour.<enviso-notice id="custom-parking-button" icon-name="sorting-arrows-horizontal" name="Mark your parking spot"> Add a parking ticket to your basket now and get a 5% discount! <enviso-button slot="action" onclick="addParkingTicket()">Add a parking ticket</enviso-button> </enviso-notice>
Define in the script the
offerId
and theproductId
.const parkingOfferId = *your offer ID*; const parkingProductId = *your ticket ID*;
Add the
addParkingTicket()
codeto the script. This code first creates a new reservation for the given ticket. In this function, a discount code will be added to your basket, which gives you 5% off on your parking ticket. Next, the basket is refreshed and the button is hidden. The helper functions are explained further down.async function addParkingTicket() { await enviso.apis.directSelling.createReservations( { reservations: [{ productId: parkingProductId, quantity: 1 }]}); await enviso.basket.plugins.flatMap(plugin => plugin.applyDiscount("PARKING2020")); await enviso.basket.plugins.flatMap(plugin => plugin.refresh()); hideParkingButton(); }
Logic for showing and hiding the button. In order to show the button, add the
slot
attribute to the element in the script. To hide it, remove theslot
attribute. An extra check on an empty basket is also done, so you don't show an upsell button on an empty basket. In theory, it would be possible to do this, but in most cases, customers first will add their products and then choose their parking tickets.function showHideParkingButton() { if (isOfferInBasket(parkingOfferId) || hasEmptyBasket()) { hideParkingButton(); } else { showParkingButton(); } } function hasEmptyBasket() { return !!enviso.basket && enviso.basket.getBasketItemsArray().length === 0; } function showParkingButton() { document.querySelectorAll("#custom-parking-button")[0].setAttribute('slot', 'above-basket-items'); } function hideParkingButton() { document.querySelectorAll("#custom-parking-button")[0].removeAttribute('slot'); }